List of JSData Components
The classes that make up JSData.
Tip
Everything in JSData is a class, whose behavior decomposed into many methods. Bend JSData to your will by extending, e.g.
import { Record, Container } from 'js-data'; class StudentRecord extends Record { // add a method say () { return this.name; } // override a method toJSON (opts) { if (/* some condition */) { // return something custom } // or fall back to default behavior return super.toJSON(opts); } } const store = new Container(); store.defineMapper('student', { recordClass: StudentRecord });
Component
The Component class is the base class from which all JSData components inherit. You do not need to use the Component
class directly to use JSData.
Classes that inherit from Component
receive on(), off(), and emit() methods that allow instances to act as an event bus. Records emit change events, Collections emit add and remove events, and the events of their Records. Mappers emit lifecycle events. Store emit all of the events of their constituents.
Tip
Feel free to use the
Component
class in your own code, and emit custom events on JSData components.
Classes that inherit from Component
also receive the dbg() and log() methods. By default log()
writes data to the console
, but you can override any components log()
method to pipe logging data to your own log sink. dbg()
is short for log('debug', ...)
, and will only actually log anything if the components debug
property is set to true
.
Tip
In your app, you can setup logging statements on JSData components that only log anything when you flip the switch, i.e. set
debug
totrue
.
Mapper
The Mapper class is the heart and soul of JSData. Mappers represent your Resources and define the CRUD interface. Mappers use the Adapter Pattern to delegate to Adapters which implement storage-specific functionality for the CRUD interface. Mappers use the Data Mapper Pattern to move data between your app and your persistent storage.
See part 2 of the Tutorial: Modeling your data.
Tip
It helps to think of Mappers as representations of your Resources, which typically map to tables or collections in your database.
Mappers:
- Do not hold any state (apart from their configuration), they only shuttle Records between your app and your persistence layer, reading data and persisting changes.
- Require at least one configured Adapter to perform any of the asynchronous CRUD methods.
- Emit lifecycle events, e.g. afterCreate.
- Automatically convert results to Record instances, configurable with Mapper#wrap.
- Allow you to define a Schema to aid with validation and JSONification of Records.
Adapter
The Adapter class itself is an abstract class that mirrors the CRUD interface defined by Mappers, but in general, Adapters are the storage-specific implementations of that CRUD interface. There are many official adapters in the JSData project, but anything that implements the JSData CRUD interface and is callable by a Mapper can be used as an adapter in JSData.
See part 3 of the Tutorial: Connecting to a data source.
Tip
If you're going to implement an adapter, it is recommend that you import and extend the
Adapter
class from js-data-adapter.js-data-adapter
also comes with an adapter test suite that you can use to verify your implementation.
Record
The Record class is used to represent individual data records in memory. Mappers usually take care of converting incoming data to Record
instances. You can use unsaved Record
instances in your app before persisting them to the backend.
Tip
It helps to think of Records as in-memory representations of individual rows in a table or individual documents in a collection.
Records:
- Rely on a Mapper's Schema for validation, in-memory change tracking, and JSONificiation.
- Provide a number of convenience methods, making Records "smart" according to the Active Record Pattern.
- Emit change events when the Mapper has a Schema and
track: true
is used. See Events & Listeners.
Schema
The Schema class provides an implementation for JSONSchema-compliant validation, with some JSData extensions, specifically adding active change detection for Records and secondary index creation for Collections.
See part 6 of the Tutorial: Schemas & Validation.
Tip
You can download the JSONSchema Meta-Schema and use it to validate your schemas.
Container
The Container class implements a Mapper-management system, freeing you from having to manage your Mappers manually. A Container
instance proxies Mapper methods, but require you to specify the target Mapper.
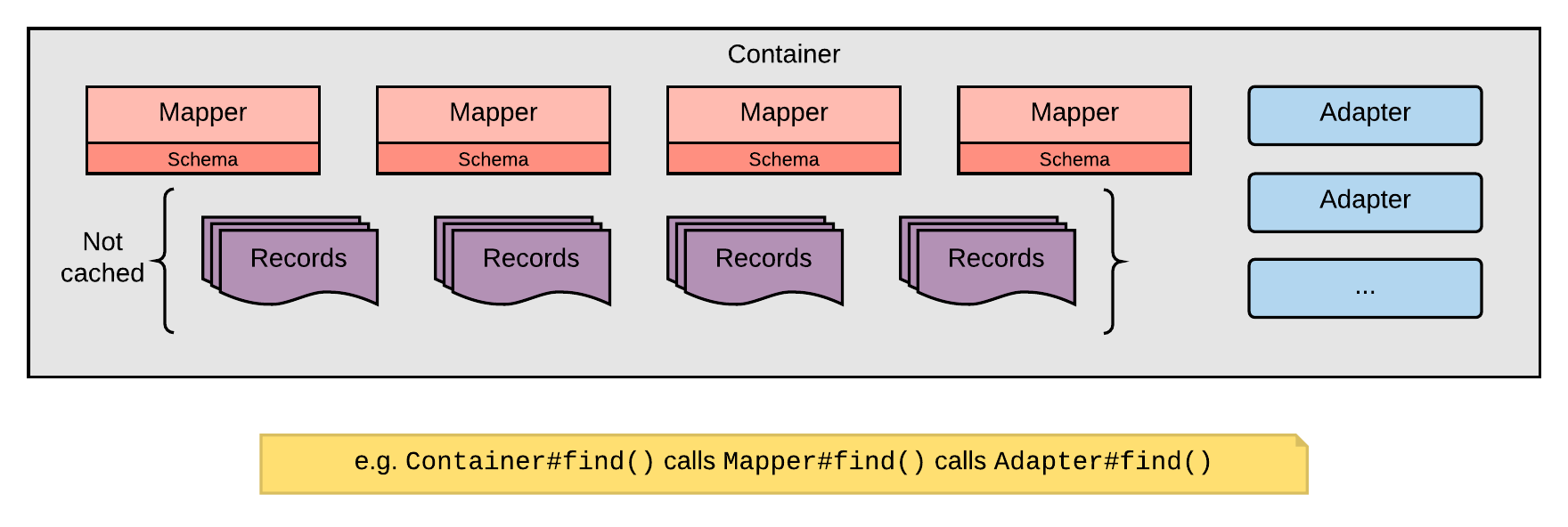
Collection
The Collection class provides an abstraction around collections of recordsβit's basically a fancy array.
Query
The Query class allows one to read data out of a Collection, including filtering, sorting, limiting, and skipping.
SimpleStore
The SimpleStore class is an opinionated, in-memory cache that utilizes Mappers, Collections, and Records to run the cache.
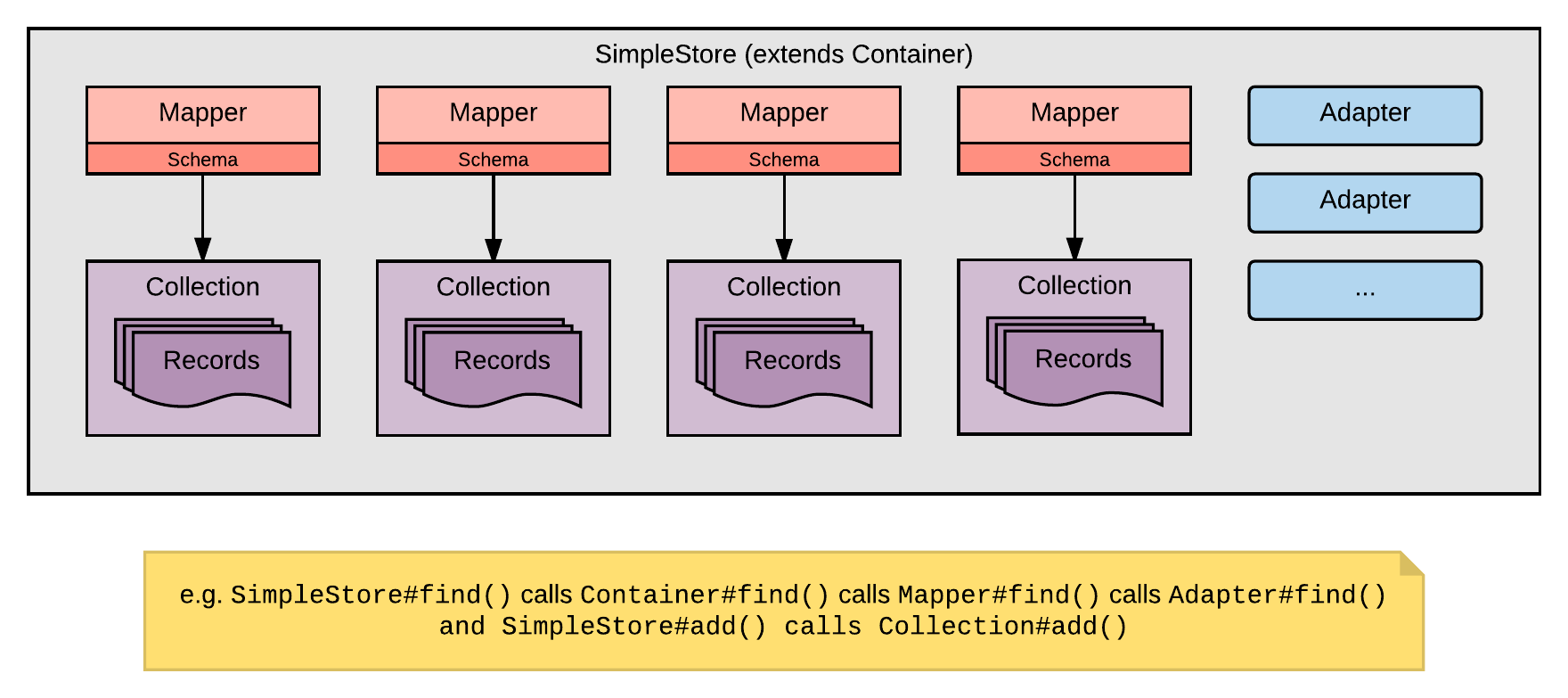
LinkedCollection
The LinkedCollection class is an extension of the Collection used by the DataStore class to implement "relation linking" within the store.
DataStore
The DataStore class is an opinionated, in-memory cache that utilizes Mappers, LinkedCollections, and Records to run the cache.
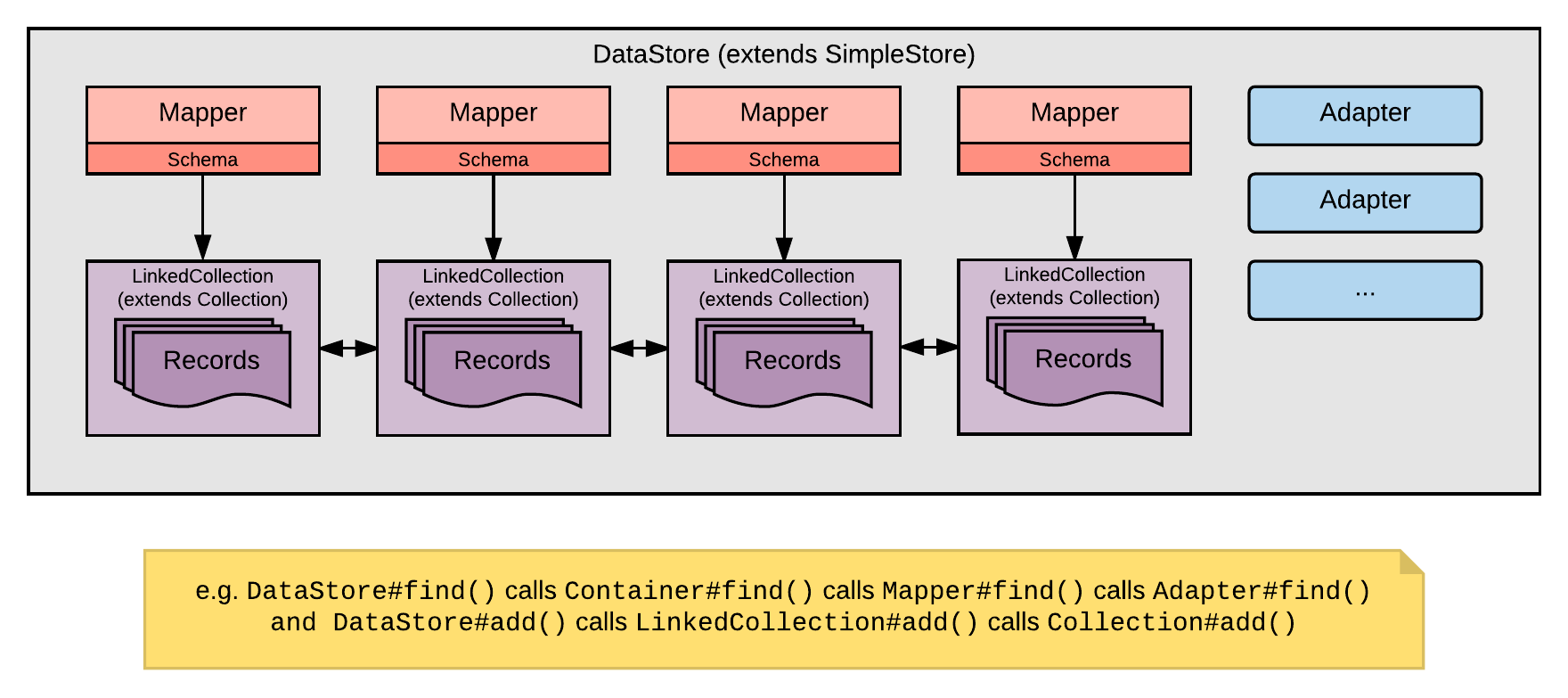
Updated over 7 years ago