Looking for the JSData v2 docs?
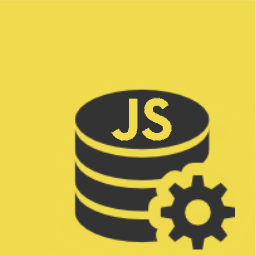
JSData is a framework-agnostic, datastore-agnostic ORM (Object-Relational Mapper) for Node.js and the Browser.
Adapters allow JSData to connect to various data sources such as Firebase, MySql, RethinkDB, MongoDB, localStorage, Redis, a REST API, etc. With JSData you can re-use your data modeling code between environments, keep your data layer intact when transitioning between app frameworks, and work with a unified data API on the server and the client. JSData employs conventions for rapid development but allows for endless customization in order to meet your particular needs.
Table of contents
Browser quick start
This example shows setting up JSData to use the Http adapter in the browser:
npm i --save js-data js-data-http
See Installation and js-data-http for more installation information.
// It is recommended to use the DataStore component in the browser
import { DataStore } from 'js-data';
import { HttpAdapter } from 'js-data-http';
const store = new DataStore();
const httpAdapter = new HttpAdapter();
// "store" will now use an HTTP adapter by default
store.registerAdapter('http', httpAdapter, { 'default': true });
// Define a Mapper for a "user" resource
store.defineMapper('user');
const runDemo = async function () {
// GET /user/1
let user = await store.find('user', 1);
console.log(user); // { id: 1, name: 'John' }
// The user record is now in the in-memory store
console.log(store.get('user', user.id)); // { id: 1, name: 'John' }
console.log(user === store.get('user', user.id)); // true
// PUT /user/1 {name:"Johnny"}
user = await store.update('user', user.id, { name: 'Johnny' });
// The user record has been updated, and the change synced to the in-memory store
console.log(store.get('user', user.id)); // { id: 1, name: 'Johnny' }
console.log(user === store.get('user', user.id)); // true
// DELETE /user/1
await store.destroy('user', user.id);
// The user record no longer in the in-memory store
console.log(store.get('user', 1)); // undefined
};
Node.js quick start
This example shows setting up JSData to use the RethinkDB adapter on the server:
npm i --save js-data js-data-rethinkdb rethinkdbdash
See Installation and js-data-rethinkdb for more installation information.
// It is recommended to use the Container component in Node.js
import { Container } from 'js-data';
import { RethinkDBAdapter } from 'js-data-rethinkdb';
const store = new Container();
const rethinkdbAdapter = new RethinkDBAdapter();
// "store" will now use a RethinkDB adapter by default
store.registerAdapter('rethinkdb', rethinkdbAdapter, { 'default': true });
// Define a Mapper for a "user" resource
store.defineMapper('user');
const runDemo = async function () {
// Find a user record in RethinkDB
let user = await store.find('user', 1);
console.log(user); // { id: 1, name: 'John' }
// Update the user record in RethinkDB
user = await store.update('user', user.id, { name: 'Johnny' });
console.log(user); // { id: 1, name: 'Johnny' }
// Delete the user record from RethinkDB
await store.destroy('user', user.id);
// The user record has been deleted from RethinkDB
};
Updated almost 7 years ago