js-data-sql
MySQL/Postgres/SQLite adapter for JSData
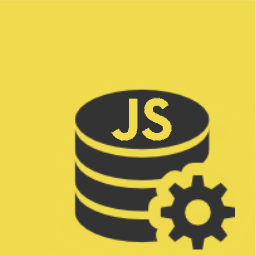
Table of contents
Installation
npm install --save js-data js-data-sql
And then you need to install one of:
pg
sqlite3
mysql
mysql2
mariasql
strong-oracle
oracle
mssql
Quick Start
This example shows how to use js-data-sql
with MySQL:
npm install --save js-data js-data-sql mysql
Here's a small sample app:
import express from 'express';
import store from './store';
const app = express();
app.route('/posts')
.post('/', async function (req, res) {
res.send(await store.create('post', req.body));
})
.get('/:id', async function (req, res) {
res.send(await store.find('post', req.params.id));
})
.put('/:id', async function (req, res) {
res.send(await store.update('post', req.params.id, req.body));
})
.delete('/:id', async function (req, res) {
res.send(await store.destroy('post', req.params.id));
})
app.listen(3000, function () {
console.log('Example app listening on port 3000!');
});
import { SqlAdapter } from 'js-data-sql';
// Create an instance of SqlAdapter
export default const adapter = new SqlAdapter({
knexOpts: {
client: 'mysql'
}
});
// Use Container instead of DataStore on the server
import { Container } from 'js-data';
import adapter from './adapter';
// Create a store to hold your Mappers
export default const store = new Container();
// Mappers in "store" will use the Sql adapter by default
store.registerAdapter('sql', adapter, { 'default': true });
store.defineMapper('post');
Configuring the adapter
More configuration details available in the API docs:
import { Container } from 'js-data';
import { SqlAdapter } from 'js-data-sql';
const store = new Container();
const sqlAdapter = new SqlAdapter({
knexOpts: {
client: 'mysql',
connection: {
host: 'localhost',
user: 'test',
password: '123456',
database: 'foo',
port: '3306'
}
}
});
store.registerAdapter('sql', sqlAdapter, { 'default': true });
for connecting to another database see knexjs config options
Useful Links
See an issue with this tutorial?
You can open an issue or better yet, suggest edits right on this page.
Need support?
Have a technical question? Post on the JSData Stack Overflow channel.
Want to chat with the community? Hop onto the JSData Slack channel.
Updated almost 6 years ago