js-data-mongodb
MongoDB adapter for JSData on Node.js
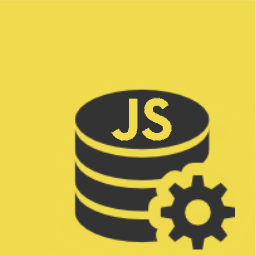
Table of contents
Installation
npm install --save js-data js-data-mongodb bson mongodb
Quick Start
Here's a small sample app:
import express from 'express';
import store from './store';
const app = express();
app.route('/posts')
.post('/', async function (req, res) {
res.send(await store.create('post', req.body));
})
.get('/:id', async function (req, res) {
res.send(await store.find('post', req.params.id));
})
.put('/:id', async function (req, res) {
res.send(await store.update('post', req.params.id, req.body));
})
.delete('/:id', async function (req, res) {
res.send(await store.destroy('post', req.params.id));
});
app.listen(3000, function () {
console.log('Example app listening on port 3000!');
});
import { MongoDBAdapter } from 'js-data-mongodb';
// Create an instance of MongoDBAdapter
export default const adapter = new MongoDBAdapter({
uri: 'mongodb://localhost:27017'
});
// Use Container instead of DataStore on the server
import { Container } from 'js-data';
import adapter from './adapter';
// Create a store to hold your Mappers
export default const store = new Container({
mapperDefaults: {
idAttribute: '_id'
}
});
// Mappers in "store" will use the RethinkDB adapter by default
store.registerAdapter('mongodb', adapter, { 'default': true });
store.defineMapper('post', {
collection: 'posts'
});
Configuring the adapter
More configuration details available in the API docs:
import { MongoDBAdapter } from 'js-data-mongodb';
// Create an instance of MongoDBAdapter
export default const adapter = new MongoDBAdapter({
debug: false, // optional - show debugging info
uri: 'mongodb://localhost:27017'
});
Custom queries
The node-mongodb-native
instance is available via a Promise on an instantiated adapter:
<Promise> = adapter.client
import { MongoDBAdapter } from 'js-data-mongodb';
const adapter = new MongoDBAdapter({
debug: true, // optional - show debugging info
uri: 'mongodb://localhost:27017'
});
// run custom query on the MongoDBAdapter
adapter
.client
.then((db) => {
// Get the documents collection
const collection = db.collection('documents');
// Insert some documents
collection.insertMany([
{a : 1}, {a : 2}, {a : 3}
], (err, result) => {
console.log(results);
});
})
.catch(err => {
// catch err
});
Useful Links
See an issue with this tutorial?
You can open an issue or better yet, suggest edits right on this page.
Need support?
Have a technical question? Post on the JSData Stack Overflow channel.
Want to chat with the community? Hop onto the JSData Slack channel.
Updated almost 7 years ago