js-data-rethinkdb
RethinkDB adapter for JSData
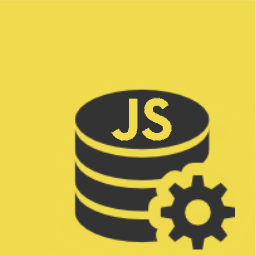
Table of contents
Installation
npm install --save js-data js-data-rethinkdb rethinkdbdash
Quick Start
Here's a small sample app:
import express from 'express';
import store from './store';
const app = express();
app.route('/posts')
.post('/', async function (req, res) {
res.send(await store.create('post', req.body));
})
.get('/:id', async function (req, res) {
res.send(await store.find('post', req.params.id));
})
.put('/:id', async function (req, res) {
res.send(await store.update('post', req.params.id, req.body));
})
.delete('/:id', async function (req, res) {
res.send(await store.destroy('post', req.params.id));
});
app.listen(3000, function () {
console.log('Example app listening on port 3000!');
});
import { RethinkDBAdapter } from 'js-data-rethinkdb';
// Create an instance of RethinkDBAdapter
export default const adapter = new RethinkDBAdapter({
rOpts: {
host: process.env.DB_HOST,
port: process.env.DB_POST,
db: process.env.DB_DATABASE,
authKey: process.env.DB_AUTH_KEY
}
});
// Use Container instead of DataStore on the server
import { Container } from 'js-data';
import adapter from './adapter';
// Create a store to hold your Mappers
export default const store = new Container();
// Mappers in "store" will use the RethinkDB adapter by default
store.registerAdapter('rethinkdb', adapter, { default: true });
store.defineMapper('post');
Configuring the adapter
More configuration details available in the API docs:
import { RethinkDBAdapter } from 'js-data-rethinkdb';
// Create an instance of RethinkDBAdapter
export default const adapter = new RethinkDBAdapter({
// Pass config to rethinkdbdash here
rOpts: {...}
});
Custom queries
Each instance of RethinkDBAdapter
creates an instance of rethinkdbdash
—the client that connects to RethinkDB. This client is available at RethinkDBAdapter#r
.
If you need to manually execute any query you can do so using the r
client attached to the instance, for example:
const indexList = await adapter.r.table('someTable').indexList().run();
adapter
.r
.db('someDb')
.table('someTable')
.indexList()
.run()
.then(function (indexList) {
// ...
});
You can also take any RQL stream and apply js-data's filtering/sorting/limiting/offseting syntax to it, for example:
const numPublished = await adapter
.filterSequence(adapter.r.table('post'), { published: true })
.count()
.run();
adapter.filterSequence(
adapter.r.table('post'),
{ published: true }
).count().run().then(function (numPublished) {
// ...
})
Useful Links
See an issue with this tutorial?
You can open an issue or better yet, suggest edits right on this page.
Need support?
Have a technical question? Post on the JSData Stack Overflow channel.
Want to chat with the community? Hop onto the JSData Slack channel.
Updated over 7 years ago