js-data-firebase
Firebase adapter for JSData
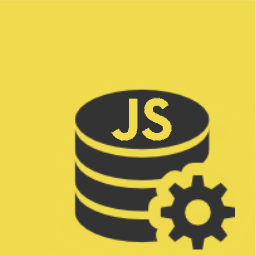
Table of contents
Installation
npm install --save js-data js-data-firebase base
Quick Start
Here's a small sample for using js-data-firebase
in the browser:
window.firebase.initializeApp({
apiKey: window.API_KEY,
authDomain: window.AUTH_DOMAIN,
databaseURL: window.DATABASE_URL
});
import store from './store';
store.find('post', 1234).then((post) => {
console.log(post);
});
import { FirebaseAdapter } from 'js-data-firebase';
// Create an instance of FirebaseAdapter
export default const adapter = new FirebaseAdapter({
db: window.firebase.database()
});
// Use DataStore instead of Container in the browser
import { DataStore } from 'js-data';
import adapter from './adapter';
// Create a store to hold your Mappers
export default const store = new DataStore();
// Mappers in "store" will use the Firebase adapter by default
store.registerAdapter('firebase', adapter, { default: true });
store.defineMapper('post');
Here's a small sample for using js-data-firebase
in Node.js:
const firebase = require('firebase);
firebase.initializeApp({
databaseURL: process.env.DATABASE_URL,
serviceAccount: process.env.KEY_FILENAME || 'key.json'
});
import express from 'express';
import store from './store';
const app = express();
app.route('/posts')
.post('/', async function (req, res) {
res.send(await store.create('post', req.body));
})
.get('/:id', async function (req, res) {
res.send(await store.find('post', req.params.id));
})
.put('/:id', async function (req, res) {
res.send(await store.update('post', req.params.id, req.body));
})
.delete('/:id', async function (req, res) {
res.send(await store.destroy('post', req.params.id));
});
app.listen(3000, function () {
console.log('Example app listening on port 3000!');
});
const firebase = require('firebase');
import { FirebaseAdapter } from 'js-data-firebase';
// Create an instance of FirebaseAdapter
export default const adapter = new FirebaseAdapter({
db: firebase.database()
});
// Use Container instead of DataStore in Node.js
import { Container } from 'js-data';
import adapter from './adapter';
// Create a store to hold your Mappers
export default const store = new Container();
// Mappers in "store" will use the Firebase adapter by default
store.registerAdapter('firebase', adapter, { default: true });
store.defineMapper('post');
Configuring the adapter
TODO
Useful Links
See an issue with this tutorial?
You can open an issue or better yet, suggest edits right on this page.
Need support?
Have a technical question? Post on the JSData Stack Overflow channel.
Want to chat with the community? Hop onto the JSData Slack channel.
Updated over 7 years ago