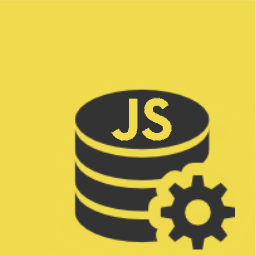
js-data-documentdb
is an Azure DocumentDB adapter for JSData.
Table of contents
Quick Start
npm install --save documentdb js-data@rc js-data-documentdb@rc
import { Container } from 'js-data';
import { DocumentDBAdapter } from 'js-data-documentdb';
const docAdapter = new DocumentDBAdapter({
documentOpts: {
// Store data in a DocumentDM database called "mydb"
db: 'mydb',
urlConnection: process.env.DOCUMENT_DB_ENDPOINT,
auth: {
masterKey: process.env.DOCUMENT_DB_KEY
}
}
});
const store = new Container();
store.registerAdapter('documentdb', docAdapter, { 'default': true });
store.defineMapper('school', {
// Store data in a DocumentDB "schools" collection
collection: 'schools'
});
store.defineMapper('student', {
// Store data in a DocumentDB "students" collection
collection: 'students'
});
// DocumentClient#readDocument(docLink, ...)
store.find('school', 1).then((school) => {
console.log('school');
});
Dependencies
Has a peer dependency on documentdb.
See also JSData's dependencies.
Configuring the adapter
The JSData DocumentDB adapter can be configured upon instantiation and anytime thereafter. See the configuration options
import { DocumentDBAdapter } from 'js-data-documentdb';
const options = {
documentOpts: {
db: 'mydb',
urlConnection: process.env.DOCUMENT_DB_ENDPOINT,
auth: {
masterKey: process.env.DOCUMENT_DB_KEY
}
}
};
// pass options to the constructor
const docAdapter = new DocumentDBAdapter(options);
console.log(docAdapter.documentOpts.db); // "mydb"
docAdapter.documentOpts.db = 'otherdb';
console.log(docAdapter.documentOpts.db); // "otherdb"
Extending the adapter
The options
arguments passed to the DocumentDBAdapter
constructor function is mixed directly into the new instance. This allows you to extend the adapter during construction:
import { DocumentDBAdapter } from 'js-data-documentdb';
const options = {
documentOpts: {
db: 'mydb',
urlConnection: process.env.DOCUMENT_DB_ENDPOINT,
auth: {
masterKey: process.env.DOCUMENT_DB_KEY
}
},
find: function (mapper, id, opts) {
if (/* some condition */) {
// do something custom
}
// Else, do default behavior
return DocumentDBAdapter.prototype.find.call(this, mapper, id, opts);
}
};
// pass options to the constructor
const docAdapter = new DocumentDBAdapter(options);
You can also extend the DocumentDBAdapter
class:
import { DocumentDBAdapter } from 'js-data-documentdb';
class CustomDocumentDBAdapter extends DocumentDBAdapter {
find(mapper, id, opts) {
if (/* some condition */) {
// do something custom
}
// Else, do default behavior
return super.find(mapper, id, opts);
}
}
const options = {
documentOpts: {
db: 'mydb',
urlConnection: process.env.DOCUMENT_DB_ENDPOINT,
auth: {
masterKey: process.env.DOCUMENT_DB_KEY
}
}
};
// pass options to the constructor
const docAdapter = new CustomDocumentDBAdapter(options);
var DocumentDBAdapter = require('js-data-documentdb').DocumentDBAdapter;
var CustomDocumentDBAdapter = DocumentDBAdapter.extend({
find: function (mapper, id, opts) {
if (/* some condition */) {
// do something custom
}
// Else, do default behavior
return DocumentDBAdapter.prototype.find.call(this, mapper, id, opts);
}
});
var options = {
documentOpts: {
db: 'mydb',
urlConnection: process.env.DOCUMENT_DB_ENDPOINT,
auth: {
masterKey: process.env.DOCUMENT_DB_KEY
}
}
};
// pass options to the constructor
var docAdapter = new CustomDocumentDBAdapter(options);
Custom queries
The DocumentDB adapter uses in instance of DocumentClient
to communicate with Azure DocumentDB. You can access this client and use it to perform custom queries:
import { Container } from 'js-data';
import { DocumentDBAdapter } from 'js-data-documentdb';
const store = new Container();
store.defineMapper('book', {
collection: 'books'
});
const options = {
documentOpts: {
db: 'mydb',
urlConnection: process.env.DOCUMENT_DB_ENDPOINT,
auth: {
masterKey: process.env.DOCUMENT_DB_KEY
}
}
};
// pass options to the constructor
const docAdapter = new DocumentDBAdapter(options);
const client = docAdapter.client;
const booksLink = docAdapter.getCollectionLink(store.getMapper('book'));
const querySpec = {
query: 'SELECT * from books'
};
client.queryDocuments(docAdapter, querySpec, (err, books) => {
console.log(books);
});
Using the HTTP adapter's lifecycle hooks
Like JSData itself, the DocumentDB adapter has lifecycle hooks. You can find them enumerated in the API Reference Documentation. The DocumentDB adapter has the same CRUD lifecycle hooks as JSData.
Here's an example of overriding the afterFindAll
:
import { DocumentDBAdapter } from 'js-data-documentdb';
const options = {
documentOpts: {
db: 'mydb',
urlConnection: process.env.DOCUMENT_DB_ENDPOINT,
auth: {
masterKey: process.env.DOCUMENT_DB_KEY
}
},
afterFindAll: function (mapper, query, opts, result) {
if (/* some condition */) {
// do something custom
}
// Now do the default behavior
return DocumentDBAdapter.prototype.afterFindAll.call(this, mapper, query, opts, result);
}
};
// pass options to the constructor
const docAdapter = new DocumentDBAdapter(options);
import { DocumentDBAdapter } from 'js-data-http';
class CustomDocumentDBAdapter extends DocumentDBAdapter {
afterFindAll(mapper, query, opts, result) {
if (/* some condition */) {
// do something custom
}
// Now do the default behavior
return super.afterFindAll(mapper, query, opts, result);
}
}
const options = {
documentOpts: {
db: 'mydb',
urlConnection: process.env.DOCUMENT_DB_ENDPOINT,
auth: {
masterKey: process.env.DOCUMENT_DB_KEY
}
}
};
// pass options to the constructor
const docAdapter = new CustomDocumentDBAdapter(options);
Links
See an issue with this tutorial?
You can open an issue or better yet, suggest edits right on this page.
Need support?
Have a technical question? Post on the JSData Stack Overflow channel or the Mailing list.
Want to chat with the community? Hop onto the JSData Slack channel.
Updated less than a minute ago